Microservice architecture enables developers to build scalable, resilient, and independently deployable services. When combined with Docker, microservices become even more manageable, portable, and efficient.
This post will cover Docker and microservice architecture and how the two integrate to create efficient, maintainable systems. Additionally, you'll learn actionable steps for building a Docker-based microservice architecture, addressing challenges, and following design principles.
What Is Docker?
Docker is an open-source platform designed to automate the deployment, scaling, and management of applications using containerization. Containers are lightweight, stand-alone, and executable packages that include everything an application needs to run, such as code, libraries, dependencies, and system tools. Moreover, Docker allows developers to bundle code, dependencies, and configurations into containers, ensuring consistency across development and production environments.
Unlike traditional virtual machines, containers share the host operating system's kernel, making them faster and more resource-efficient. Docker provides tools like the Docker Engine for building and running containers. Furthermore, it provides Docker Compose for orchestrating multicontainer applications and Docker Hub for managing container images.
Key Benefits of Docker
- Portability: Run applications anywhere—on-premises or in the cloud. Containers ensure that the same application works seamlessly across different environments.
- Scalability: Easily scale applications by spinning up or down containers, enabling systems to handle varying loads effectively.
- Resource efficiency: Containers share the host system's kernel, making them more efficient compared with traditional virtual machines, which require separate operating systems.
- Consistent development workflow: Docker eliminates the "it works on my machine" problem by ensuring identical environments across development, testing, and production.
What Is Microservice Architecture?
Microservice architecture is a design approach where applications are composed of small, independent services, each focused on a specific business function. These services communicate through APIs, allowing developers to build, deploy, and scale components independently.
Microservices contrast monolithic architecture, where all functionalities are bundled together. While monolithic systems can be simpler initially, they often become difficult to scale and maintain as they grow.
Core Principles of Microservice Architecture
- Decentralization: Services operate independently and are developed by separate teams, reducing bottlenecks and promoting autonomy.
- Resilience: Failure in one service doesn’t bring down the entire application. Techniques like retries, fallbacks, and circuit breakers enhance fault tolerance.
- Scalability: Services can be scaled independently based on demand, optimizing resource allocation.
- Technology agnosticism: Teams can choose the best technology stack for each service, enabling innovation and flexibility.
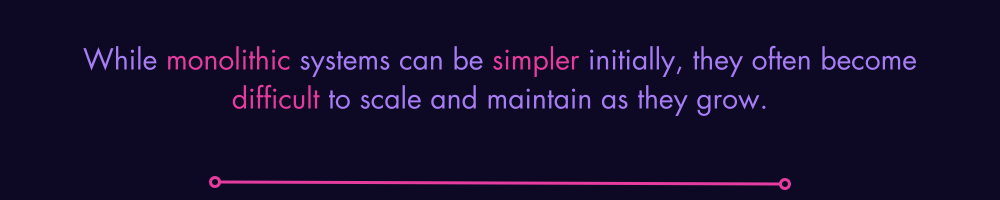
How to Deploy Microservice Architecture in Docker
Building and deploying a microservice architecture in Docker involves a systematic approach, addressing key considerations such as breaking down monolithic applications, designing efficient microservices, and utilizing Docker’s capabilities.
This section walks you through the process step by step.
Breaking Down a Monolith
The first step in transitioning to microservices is identifying and separating functionalities within a monolithic application. Follow these steps to break down a monolith:
1. Identify Functional Boundaries
Begin by analyzing your monolithic application to identify distinct business functionalities. Use tools like domain-driven design (DDD) to map out domains and determine which parts of the application can be logically separated.
2. Group Functionality Into Services
Once functionalities are identified, group-related components (e.g., APIs, database schemas) are divided into smaller, self-contained units that will later become individual microservices.
3. Create Independent Services
Reimplement each group as an independent service. For example, in an e-commerce system, the order processing, inventory, and user management modules could each become separate microservices. Ensure each service has its database to maintain independence.
4. Decouple Data
Avoid shared databases between microservices. Additionally, use APIs or message brokers for inter-service communication to ensure loose coupling and autonomy.
Challenges of Building a Microservice Architecture
While microservices offer benefits, they introduce challenges such as:
- Increased complexity—Managing multiple independent services introduces complexity in deployment, monitoring, and debugging.
- Inter-service communication—Services need to communicate reliably. Choose between synchronous protocols like REST/gRPC or asynchronous methods like RabbitMQ/Kafka.
- Data management—Ensuring consistency and handling distributed transactions can be challenging when each service maintains its data.
- Monitoring and observability—Tracking issues across services requires implementing centralized logging (e.g., using ELK Stack) and distributed tracing (e.g., Jaeger).
How Docker Helps in Building a Microservice Architecture
Docker simplifies microservice deployment by providing:
- Isolation of services—Each service is encapsulated within its own Docker container, avoiding dependency conflicts and ensuring consistent runtime environments.
- Portability across environments—Docker ensures that services run identically in development, testing, and production, eliminating "it works on my machine" issues.
- Scalable deployments—Docker’s lightweight containers allow you to spin up multiple service instances to handle increased demand.
- Ease of testing—Docker makes it easy to replicate production-like environments locally for testing individual services or the entire system.
Principles of Designing an Efficient Microservice Architecture
To design robust microservices, follow these principles:
- Single responsibility principle—Each microservice should handle one specific functionality. For example, a payment service should focus solely on payment processing.
- API-first design—Define clear, versioned APIs to facilitate communication between services. Use OpenAPI/Swagger for API documentation.
- Resilience and fault tolerance—Implement retries, timeouts, and circuit breakers to handle failures gracefully and avoid cascading issues.
- Decentralized data management—Each service should own its data and database. For example, customer service manages customer-related data while the order service manages order records.
Step-by-Step Guide to Deploy Microservice Architecture in Docker
Follow these steps to deploy a microservice architecture in Docker.
Step 1: Break Down Your Application into Microservices
First, divide the application into smaller, independent services, each responsible for a specific business capability. For example, a typical e-commerce app may include microservices for user management, catalog, orders, and payments. Each microservice should have its repository, build process, and deployment life cycle.
Step 2: Install Docker and Docker Compose
Next, ensure that Docker is installed on your system and that Docker Compose is available for managing multi-container setups.
# Install Docker
sudo apt update
sudo apt install docker.io
# Install Docker Compose
sudo curl -L "https://github.com/docker/compose/releases/latest/download/docker-compose-$(uname -s)-$(uname -m)" -o /usr/local/bin/docker-compose
sudo chmod +x /usr/local/bin/docker-compose
Step 3: Set Up the Directory Structure
After that, organize your microservices into directories. For example:
project-root/
├── service-a/
│ ├── Dockerfile
│ └── app/
├── service-b/
│ ├── Dockerfile
│ └── app/
└── docker-compose.yml
Step 4: Containerize Each Microservice
Use Docker to containerize each microservice. Create a Dockerfile for every service, specifying the dependencies, runtime, and build instructions.
Example Dockerfile:
FROM python:3.9-slim
WORKDIR /app
COPY . .
RUN pip install -r requirements.txt
CMD ["python", "app.py"]
This approach ensures that each service runs in an isolated environment, making it portable and consistent across different systems. You can then run the following commands to build the Docker image and run the container:
Build the Docker Image
docker build -t <my-service-name> .
Run the Container
docker run -d -p 5000:5000 --name <my-service-name> <my-service-name>
Step 5: Define Your docker-compose.yml File
After that, create a docker-compose.yml file to define and manage multiple services.
Example docker-compose.yml file:
version: '3.8'
services:
user-service:
build:
context: ./service-a
ports:
- "5000:5000"
order-service:
build:
context: ./service-b
ports:
- "5001:5001"
This file simplifies multi-container orchestration and allows you to specify dependencies, networks, and environment variables for the services.
Run the services with:
docker-compose up -d
Step 6: Implement Data Persistence
Ensure each microservice uses its database instance to avoid tight coupling. Use Docker volumes to persist data:
services:
user-service:
volumes:
- user-data:/var/lib/postgresql/data
volumes:
user-data:
When you isolate databases, each service can evolve independently without affecting others.
Step 7: Set Up Service Discovery and Load Balancing
Service discovery ensures that microservices can find and communicate with each other dynamically. Use Docker's internal DNS to resolve service names defined in the docker-compose.yml file. Additionally, incorporate a load balancer like Traefik or NGINX to distribute traffic evenly.
Example Traefik configuration:
labels:
- "traefik.http.services.user-service.loadbalancer.server.port=5000"
- "traefik.http.routers.user-service.rule=Host(`users.example.com`)"
Step 8: Configure Service Communication
Effective communication between microservices is crucial for a functional microservice architecture. Docker Compose automatically sets up a private network for the containers, allowing services to communicate via service names defined in the docker-compose.yml file. Follow these steps to configure service communication:
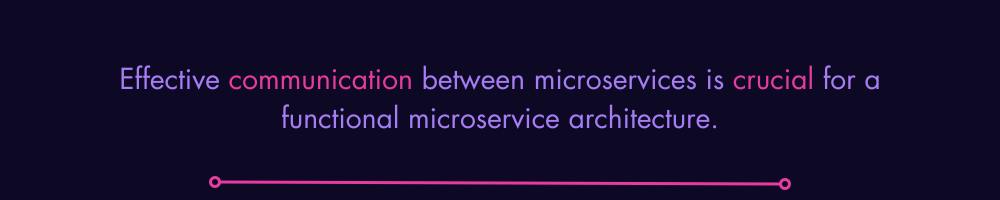
1. Define Service Names in docker-compose.yml
When services are defined in docker-compose.yml, Docker assigns each service a host name based on its name. These host names act as DNS entries, enabling communication between services.
Example:
services:
service-a:
build: ./service-a
ports:
- "5000:5000"
service-b:
build: ./service-b
depends_on:
- service-a
ports:
- "5001:5001"
In this setup, service-b can communicate with service-a using http://service-a:5000.
2. Use Environment Variables for Flexibility
Define environment variables in the docker-compose.yml file to manage communication endpoints dynamically.
services:
service-a:
environment:
- PORT=5000
service-b:
environment:
- SERVICE_A_URL=http://service-a:5000
You'll eventually access these variables in the application code to establish communication:
import os
import requests
service_a_url = os.getenv('SERVICE_A_URL')
response = requests.get(f'{service_a_url}/api/resource')
3. Expose Ports and Test Connectivity
Ensure each service exposes the required ports in the docker-compose.yml file. Test communication using tools like curl or ping:
docker exec -it service-b-container-name sh
curl http://service-a:5000
4. Configure Dependencies With depends_on
The depends_on directive ensures services start in the correct order, although health checks may still be required to confirm readiness.
5. Advanced Network Configuration (Optional)
Afterward, you can use custom Docker networks to isolate or secure services as needed:
networks:
backend:
services:
service-a:
networks:
- backend
service-b:
networks:
- backend
In summary, these steps enable secure, flexible, and efficient communication between microservices in a Docker environment.
Step 9: Monitor and Debug
Finally, use Docker logs for debugging individual services:
sudo docker logs service-a
Use monitoring tools like Prometheus (metrics) and Grafana (visualization) for large-scale setups.
Here's an example:
Add Prometheus to Docker Compose
services:
prometheus:
image: prom/prometheus
ports:
- "9090:9090"
How Docker Contributes to Continuous Integration and Deployment (CI/CD)
Docker enables CI/CD workflows by providing:
- Consistent build environments—Docker ensures the application runs the same way across CI/CD pipelines.
- Seamless integration with CI/CD tools—Tools like Jenkins, GitHub Actions, and GitLab CI can build and deploy Docker containers as part of automated workflows.
- Containerized testing—Run tests in isolated environments to avoid dependency conflicts.
How Docker Simplifies Container Management
Docker simplifies container management in the following ways:
- Networking—Docker Compose sets up container networking automatically, allowing services to communicate without manual configuration.
- Scaling—Scale services dynamically using Docker Compose. For example:
sudo docker-compose up --scale service-a=3
- Resource isolation—Docker isolates service resources (e.g., CPU, memory) to prevent one service from hogging system resources.
How Docker Aids in Software Testing
Docker aids in software testing in the following ways:
- Reproducible environments—Developers can create containers that mimic production for accurate testing.
- Automated testing pipelines—Docker images can include test suites, enabling automated testing during container builds.
- Fault injection testing—Simulate failures by manipulating container behaviors (e.g., stopping a container) to test resilience.
Tools That Complement Docker
- Kubernetes: For orchestrating large-scale containerized applications with advanced features like auto-scaling and self-healing.
- Traefik: A dynamic reverse proxy for routing requests to the appropriate services in a microservice architecture.
- ELK Stack: For centralized logging and monitoring of Dockerized services.
- Helm: This tool simplifies Kubernetes configurations and deployment management.
Advantages of Docker for Microservices
Docker provides several benefits, including:
- Scalability—Easily scale services by running multiple container instances.
- Portability—Run containers consistently across different environments.
- Isolation—Each service operates independently, reducing conflicts.
- Resource efficiency—Containers consume fewer resources than traditional virtual machines.
Why Choose DevZero for Microservice Development?
DevZero simplifies the complexities of microservice development by providing a developer-friendly platform for building, testing, and deploying applications. With DevZero, teams can
- quickly spin up consistent development environments.
- eliminate resource bottlenecks, enabling faster iteration cycles.
- reduce setup and debugging time by providing pre-configured environments.
DevZero also seamlessly integrates with Docker and Kubernetes, making it an excellent choice for organizations looking to streamline their microservices development workflows. Ready to simplify your development workflows? Explore how DevZero can transform your approach to microservices today.